Let's start with the fact that we need to create a bot that will send us messages on behalf of our flespi account.
To do this, open https://t.me/BotFather, click on the "Menu" button, select the /newbot
command, and follow the steps to create the bot.
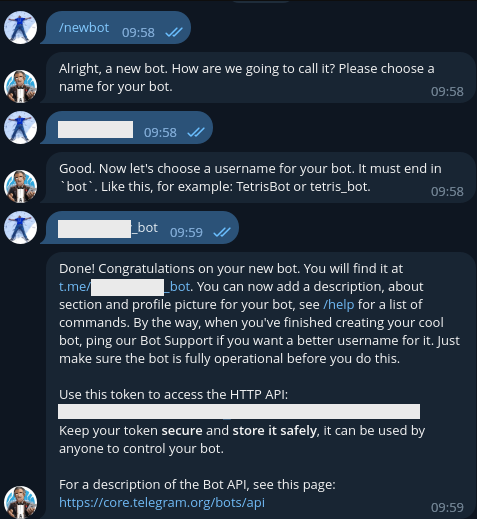
At the end, you will be provided with your bot's token.
Now we also need to obtain the chat ID.
To do this, go to the chat of the created bot and send it any message.
After that, open the following URL in your browser and find the chat ID that we need.
https://api.telegram.org/bot<YOUR_BOT_TOKEN>/getUpdates
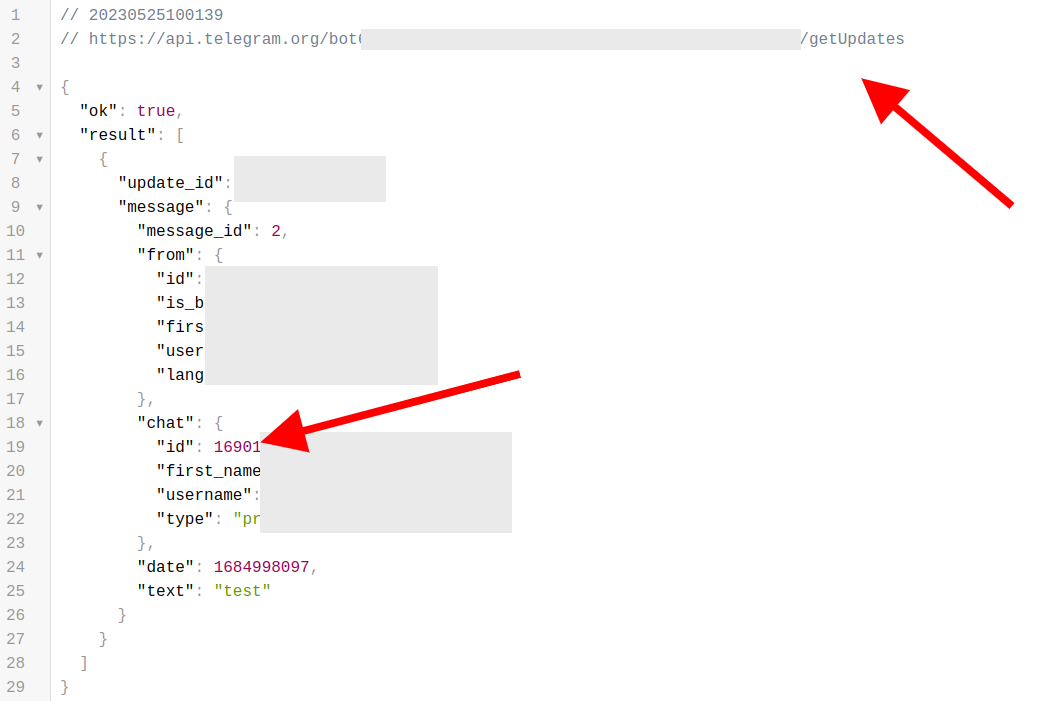
Now go to flespi.io -> Utils -> Webhooks -> "+"
Device connected/disconnected notification
Let's say we want to receive a notification when your device connects to the platform.
Create a trigger for the topic flespi/state/gw/devices/+/connected
("+" in the topic means that we will receive notifications about the connection/disconnection of all devices in the account).
Now we need to set up communication with the bot. We will use a URI of the following format:
https://api.telegram.org/bot<YOUR_BOT_TOKEN>/sendMessage
And our request body will look something like this:
{"chat_id": "<YOUR_CHAT_ID>", "text": "Device %topics[4]% connected: %payload%", "disable_notification": false}
Also, we need to add the request header Content-Type: application/json
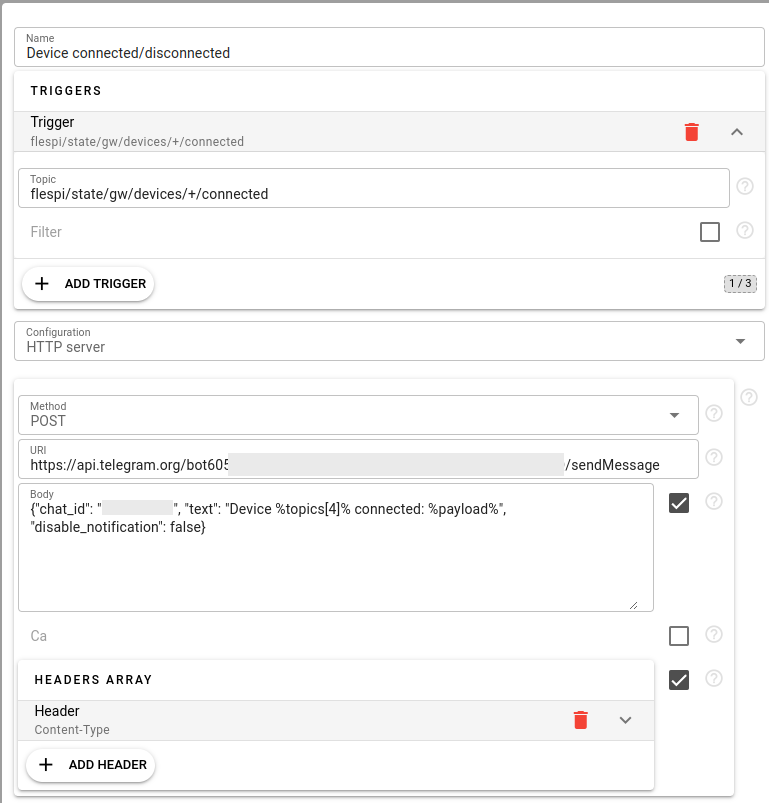
Save it and observe how notifications about device connections start coming to us.
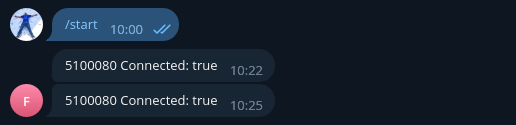
{
"triggers": [
{
"topic": "flespi/state/gw/devices/+/connected"
}
],
"enabled": true,
"name": "Device connected/disconnected",
"configuration": {
"body": "{\"chat_id\": \"<YOUR_CHAT_ID>\", \"text\": \"Device %topics[4]% connected: %payload%\", \"disable_notification\": false}",
"headers": [
{
"name": "Content-Type",
"value": "application/json"
}
],
"method": "POST",
"type": "custom-server",
"uri": "https://api.telegram.org/bot<YOUR_BOT_TOKEN>/sendMessage"
},
"queue_ttl": 86400
}
Channel/stream blocked notification
And now let's come up with a slightly more useful task. For example, we want to monitor when a channel is blocked for any reason.
Almost all the steps are similar, except for the topic (now we will subscribe to the topic of blocking all channels):
flespi/state/gw/channels/+/blocked
Let's also make the text of the message more complex with expressions:
{"chat_id": "<YOUR_CHAT_ID>", "text": "%topics[4]% blocked: %if(payload == 1, "Exceeded connections limit", if(payload == 2, "Exceeded messages limit per minute", if(payload == 3, "Exceeded traffic limit per minute", if(payload == 4, "Exceeded storage limit", if(payload == 5, "Exceeded items limit", if(payload == 6, "Item configuration is invalid", if(payload == 7, "Customer was moved to another region", if(payload==null, "unblocked", payload))))))))% https://flespi.io/#/panel/open/%topics[3]%/%topics[4]%", "disable_notification": false}
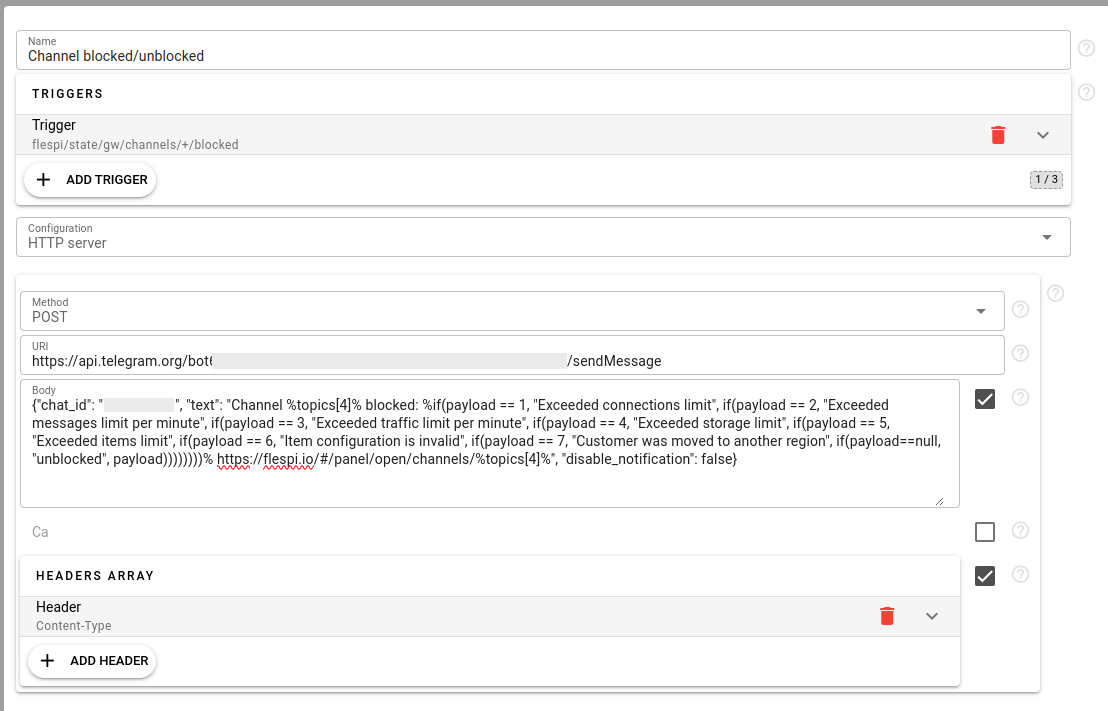
Now we have started receiving messages about channel blocking with an explanation of the reason for the blockage.
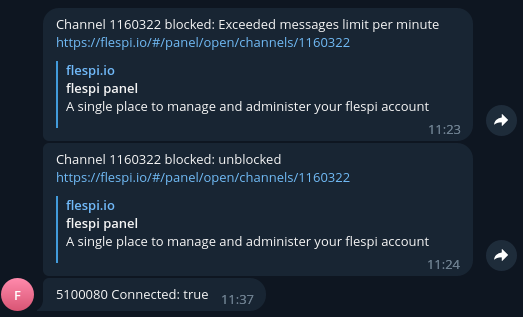
{
"triggers": [
{
"topic": "flespi/state/gw/channels/+/blocked"
},
{
"topic": "flespi/state/gw/streams/+/blocked"
}
],
"enabled": true,
"name": "Item blocked/unblocked",
"configuration": {
"body": "{\"chat_id\": \"<YOUR_CHAT_ID>\", \"text\": \"%topics[4]% blocked: %if(payload == 1, \"Exceeded connections limit\", if(payload == 2, \"Exceeded messages limit per minute\", if(payload == 3, \"Exceeded traffic limit per minute\", if(payload == 4, \"Exceeded storage limit\", if(payload == 5, \"Exceeded items limit\", if(payload == 6, \"Item configuration is invalid\", if(payload == 7, \"Customer was moved to another region\", if(payload==null, \"unblocked\", payload))))))))% https://flespi.io/#/panel/open/%topics[3]%/%topics[4]%\", \"disable_notification\": false}",
"headers": [
{
"name": "Content-Type",
"value": "application/json"
}
],
"method": "POST",
"type": "custom-server",
"uri": "https://api.telegram.org/bot<YOUR_BOT_TOKEN>/sendMessage"
},
"queue_ttl": 86400
}